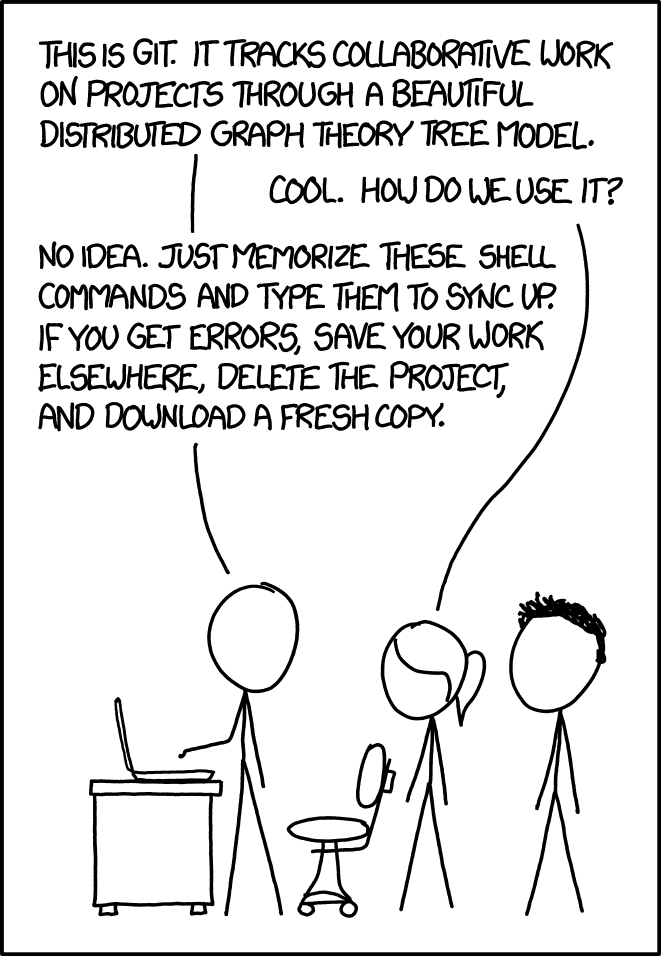
Git/GitHub and collaboration
Our old friend GitHub (and the underlying technology, Git) are robust, industry-standard tools for version control and working together on code. But to some extent, we barely scratched the surface! These are some other important Git/GitHub concepts:
- Branches
-
Besides our second project, we’ve used Git/GitHub primarily for solo, linear software development—but that is rarely how you work, especially at scale! One of Git’s core concepts is a branch (think tree metaphors). These are new/separate versions of your entire repository—which you create (people say branch, as a verb) off the
main
trunk (nobody says trunk though). When you do this to a separate repo it is called forking (people do say fork and such).Figma has started to adopt this concept, too!
- Merges
-
You might use these branches to develop a new feature, or to fix a bug, before you merge these back into
main
. Sometimes, someone else will have edited the same lines of code you were working on, in the meantime, and you’ll have to resolve a merge conflict—deciding whose work to take. (It can get messy.) - PRs (Pull Requests)
-
When you are working on big software projects/repos with multiple people—and when you don’t want to break
main
with hasty merges—you push your code up to GitHub and open a pull request (everyone says P-R). This shows all your combined commits together, and allows your team to review and approve your whole batch of work before you merge intomain
.
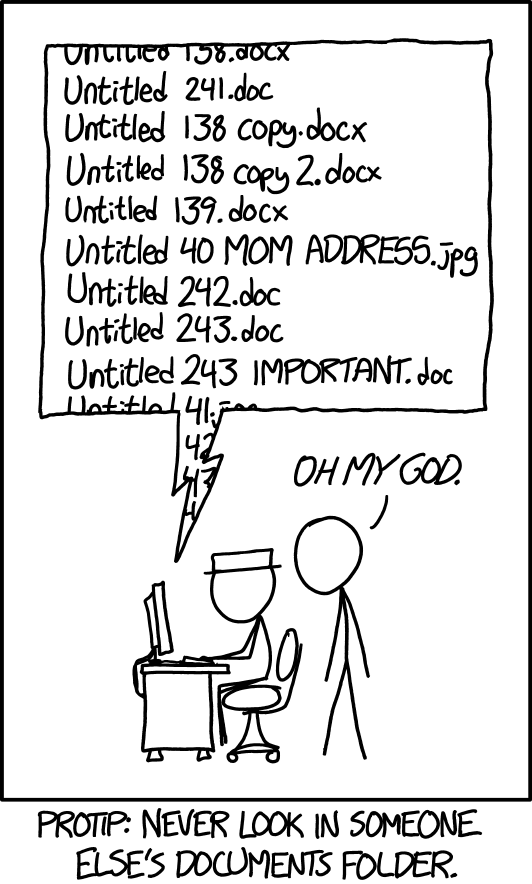
Working on software
We also only barely “dipped our toes” into project management, roadmapping, working with devs, and all the other stuff that exists around the work of making digital things. A few highlights:
- Agile
-
A popular way to structure software project management. Many product teams will use Agile methodology as a way of prioritizing tasks—working in so-called sprints or cycles (often two weeks) to deliver features incrementally rather than all at once.
One way that Agile is practiced is with a kanban board (from the Japanese), a visual way of organizing tasks into discrete phases of work so that team members understand where different stories (or tickets, tasks, or issues) are in the queue. Many tools are built around this mindset.
- Redlining / annotating
-
Often a completed design in Figma isn’t enough for handoff to a developer for implementation. There can be ambiguities in your design that you may have thought through, but need more explanation than the visual provides on its own. This could be how you expect an element to behave responsively, or maybe you have a specific dimension in mind, or you want to describe it in motion.
Redlining (not to be confused with its other meaning) or annotating is a term for when you mark up your designs with information—key measurements or other important behaviors/traits. This forms the spec that a dev should implement.
Figma’s Dev Mode is aimed at this problem!
- Bus factor / bus count
-
On small product teams, certain individuals may be the only ones who understand how a certain feature works or is implemented. The phrase bus factor (or bus count) is used in the lens of risk management—to make sure the product/team can continue if anyone gets “hit by a bus.”
Always try to avoid a bus count of one! Not just because of busses, but to distribute knowledge and understanding (and stress) among your team.
- Technical (and design) debt
-
Very often, products have to make trade-offs between design or code quality to meet deadlines, or to handle scope creep. These decisions sometimes come at the expense of either side—sacrificing the most nuanced design, or giving up on the most pristine, sturdy code. Often the short-term wins over the long-term.
Technical debt (or the analogous design debt) is the accumulation of these short-term decisions—where you have postponed doing it the right way. Like other forms of debt, these often compound over time—if you don’t pay them down or address them as you move forward.
- Dogfooding
-
Often the best way—or at least the first way—to make sure your product is up to snuff is by using and testing it yourself. This term is called dogfooding, or eating your own dog food. (There are debates on the etymology.) It’s got to be good enough for you, before it is good enough for anyone else! (Or for dogs.)
A famous example is Apple, when pushing desktop publishing in 1980, outlawing typewriters internally.
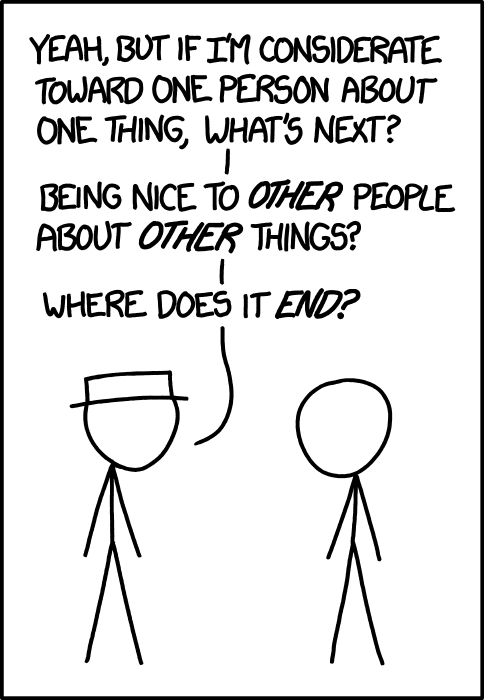
Accessibility
This is big, always-evolving topic—and one that should permeate all the others. We’ve mentioned it in passing, but know that it is a discipline unto itself and that you can be an advocate for it:
- A11y
-
A11y is a catch-all term for accessibility. This is shorthand for ensuring that your product is as inclusive as possible—for folks with different disabilities.
You might also see this kind of abbreviation for i18n, for internationalization.
- ARIA labels
-
While the best and most-basic way of writing accessible websites is to start with semantic DOM elements, sometimes there isn’t an appropriate one—this is where ARIA (Accessible Rich Internet Applications) labels come in. These are additional attributes you can add to an HTML element which help a screenreader to convey the meaning of your content:
<button aria-label="Close"><!-- A cool, close-y icon. --></button>
These are particularly important for us as designers, where our aesthetic proclivities might lean towards making our designs harder to access—by using icons, slight colors, etc.
- VoiceOver / TalkBack / Narrator
-
These are platform-specific, commonly-used assistive technologies: Apple’s VoiceOver, Google’s (Android) TalkBack, and Microsoft’s Narrator. Some disabled folks use other screen-readers, but these are a good, built-in start.
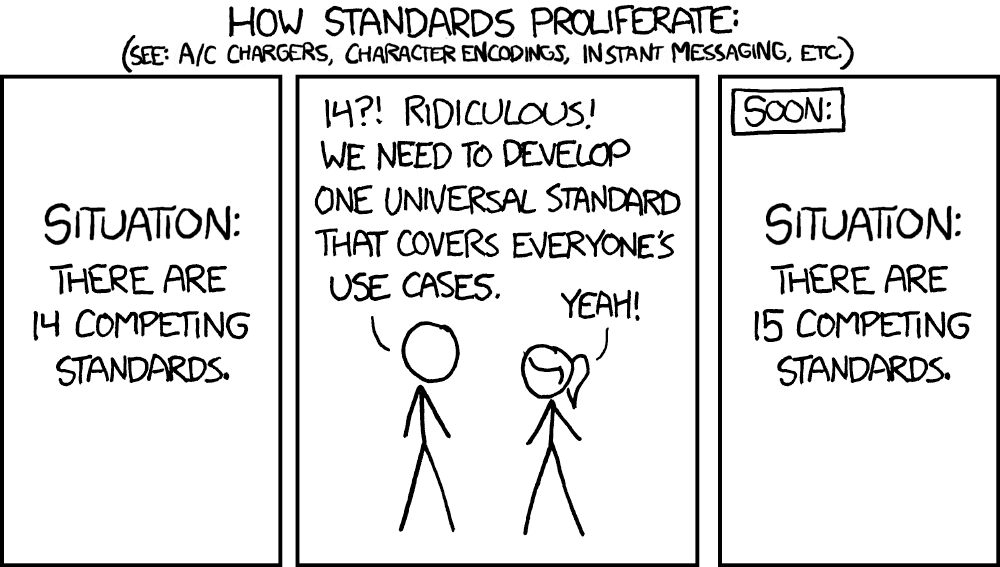
Approaches and frameworks
If you remember back to What Is Code?, software (and the web) is awash with different approaches, concepts, and opinions—and their resulting execution and frameworks. Some things worth mentioning:
- Open source / FOSS
-
We always “stand on the shoulders of giants,” and much of the web—much of all software, and so the world—is based on free and open-source software or FOSS.
This means the code is licensed such that you can use and modify it, free of charge. This is sometimes sponsored by software companies, but often is one or a few dedicated maintainers who keep the proverbial lights on.
- Multi-Page Apps (MPAs) vs. Single-Page Apps (SPAs)
-
For most of you, everything you’ve done this year could be called a multi-page app—meaning just that your websites (products) have been comprised of discrete, separate HTMLpages. The web started as simple MPA, though the term didn’t exist yet!
As the capabilities, uses, and needs of the web grew it became more app-like—with complicated interactions, changes, and state within a single “page.” Frameworks (like React or later, Vue) were created to facilitate this behavior, via JavaScript—creating a more dynamic experience within one browser session.
The line here is getting even blurrier—when you hear things like server-side rendering (SSR) and even partial hydration.
It’ll always depend on the project! But remember that at the end of the day, the browser is showing you HTML (from somewhere), styled by CSS (from somewhere), maybe enlivened by JavaScript (from somewhere). There are a lot of ways at that.
- Bootstrap
-
The framework du jour for a long time—Bootstrap was created by Twitter as a way of quickly creating web apps. Because frameworks have to have opinions to work (think back to The Web’s Grain) the downside is that many websites created using this have a certain “made with Bootstrap” vibe.
In a small environment you’ll likely be working with an existing (legacy) framework or component library, so understanding these trade-offs is important.
In CSS land…
- SASS / SCSS
-
As you may have noticed, writing CSS can be repetitive and tiresome. Back in 2006, some (very opinionated) folks developed SASS (Syntactically Awesome Style Sheets)—which is a CSS preprocessor. It is its own language/syntax (mostly based on CSS) which gets compiled (processed) into normal CSS, which your browser understands. SASS is a slightly different—but much more popular—variant of SASS. (When people say SASS, they usually mean SCSS!)
More recently, some of the utility/need for SASS has been obviated by CSS adopting similar features, like custom properties (variables) and soon, CSS nesting.
- BEM
-
You might have also noticed that coming up with CSS class names can be difficult, as well—and only gets worse as your projects get larger. BEM (Block, Element, Modifier) is a commonly-used nomenclature methodology/practice to deal with this (and also avoid specificity problems).
It follows the pattern
.block__element--modifier
in classnames—and these double separators (__
and--
) are sometimes seen even in non-BEM-y contexts. Any system is better than no system! - Tailwinds / Atomic / utility CSS
-
An alternative approach to CSS naming—following a utility class methodology. This uses many small, descriptive or directly-property-mapped names (like
.red
or.flex
) across any elements. This approach is sometimes kleenexed as atomic CSS or Tailwinds.Your instructors wrote our own atomic CSS library, baking-in/formalizing MoMA’s brand, that we called Sol.
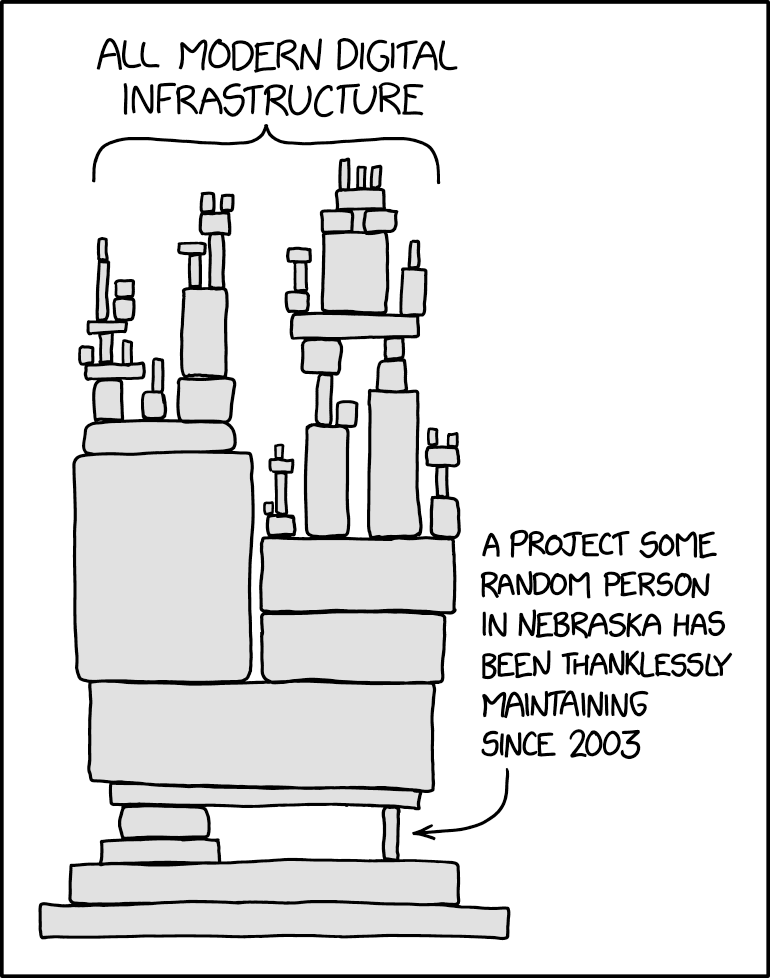